How to setup email notifications for workflow
You may want to send email notifications when an item in a workflow stage requires an action from a reviewer. Uniform provides webhooks, where you can subscribe to the 'workflow.transition' event. It looks something like this:
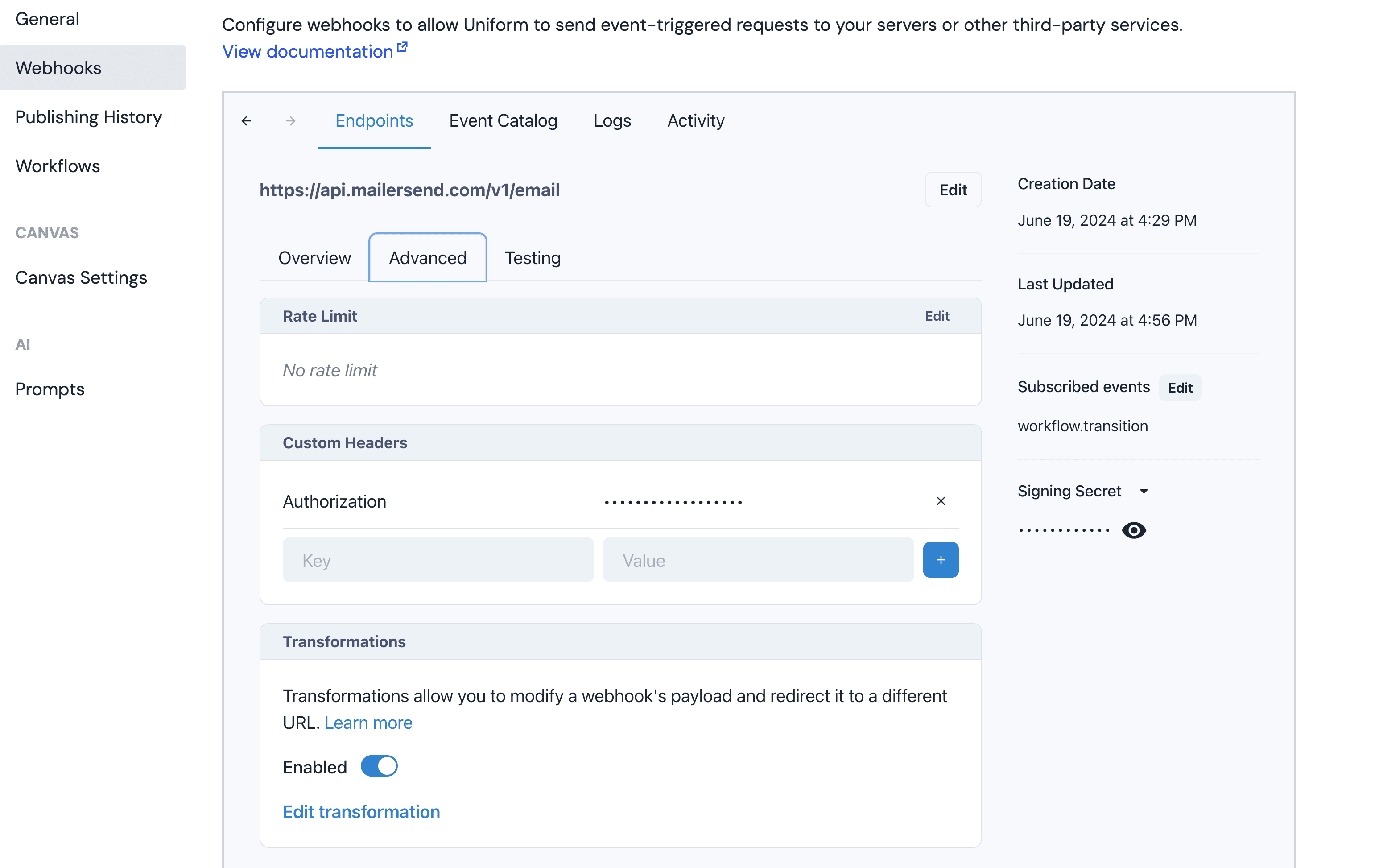
Webhook configuration example
There are services which provide API to send emails via HTTP requests. This examples is based on mailersend.com because it has a free tier, but you are welcome to use any other services.
Steps:#
- Create a webhook subscribed to the workflow.transition event and pointing to the email API, e.g. https://api.mailersend.com/v1/email
- You may need to authenticate your requests, for example via creating the API token and setting the needed Authorization header in the Custom Headers webhook section.
- Enable the webhook transformation and add a code like the one below. You need it to compose and proper email with all the details about the person triggering the workflow, stage and a link to open the content right from the email. This data is available in the webhook payload.
This is a sample code
This is a sample code, which may not cover all scenarios. Feel free to add more logic according to the specifics of the email sending service.
/**
* @param webhook the webhook object
* @param webhook.method destination method. Allowed values: "POST", "PUT"
* @param webhook.url current destination address
* @param.webhook.eventType current webhook Event Type
* @param webhook.payload JSON payload
* @param.webhook.cancel whether to cancel dispatch of the given webhook
*/
function handler(webhook) {
webhook.method = 'POST'
const subject = `Workflow notification from Uniform project`
const initiatorName = webhook?.payload?.initiator?.name ?? 'Someone'
// this line of code consider the name of the workflow stage
const isApproved = webhook?.payload?.newStage?.stageName?.includes('Approved') ?? false
const html = isApproved
? `Hi, <br /> There is a workflow notification from Uniform project<br /> ${initiatorName} has approved the ${webhook?.payload?.entity?.type} in : <br /> <a href="${webhook?.payload?.entity?.url}">${webhook?.payload?.entity?.name}</a> `
: `Hi, <br /> There is a workflow notification from Uniform project<br /> ${initiatorName} has requested approval for the ${webhook?.payload?.entity?.type} below: <br /> <a href="${webhook?.payload?.entity?.url}">${webhook?.payload?.entity?.name}</a> `
webhook.payload = {
from: {
email: 'hello@12345-mailer.mlsender.net',
name: 'MailerSend',
},
to: [
{
email: 'reviewer@yourcompany.com',
name: 'John',
},
],
subject: subject,
html: html,
personalization: [
{
email: 'reviewer@yourcompany.com',
data: {
company: 'MailerSend',
},
},
],
}
// and return it
return webhook
}