User location and device details
Akamai makes user location and device details available to EdgeWorkers. Uniform makes these details available to the classification process using quirks. Quirks, in turn, can be used to configure personalization instructions.
Identify EdgeScape data to include#
The EdgeWorker has access to Akamai EdgeScape data. Uniform is able to expose this data as quirks so it can be used in the classification process. You must specify which values you want to be exposed in this way by modifying the EdgeWorker code.
The following JavaScript code sample demonstrates how to specify several values to be exposed as quirks:
Uniform supports the following quirks:
Category | Name | Description |
---|---|---|
Device | acceptsThirdPartyCookie | true if the browser accepts third party cookies; false otherwise. |
Device | brandName | Brand name of the device. |
Device | hasAjaxSupport | true if the device supports the JavaScript functions required to make AJAX calls; false otherwise. |
Device | hasCookieSupport | true if the browser supports cookies; false otherwise. |
Device | hasFlashSupport | true if the browser supports Flash; false otherwise. |
Device | isMobile | true if the device is a mobile device; false otherwise. |
Device | isTablet | true if the device is a tablet; false otherwise. |
Device | isWireless | true if the device is a wireless device; false otherwise. |
Device | marketingName | Marketing name of the device. |
Device | mobileBrowser | Mobile browser name. |
Device | mobileBrowserVersion | Mobile browser version. |
Device | modelName | Model name of the device. |
Device | os | Device operation system. |
Device | osVersion | Device operating system version. |
Device | physicalScreenHeight | Physical screen height (in millimeters). |
Device | physicalScreenWidth | Physical screen width (in millimeters). |
Device | resolutionHeight | Screen resolution height (in pixels). |
Device | resolutionWidth | Screen resolution width (in pixels). |
Device | xhtmlSupportLevel | Browser's level of support for XHTML. |
User location | city | The city (within a 50-mile radius) that the IP address maps to. |
User location | continent | A 2-letter code that identifies the continent that the IP address maps to. |
User location | country | A 2-letter code (ISO-3166) that identifies the country that the IP address maps to. |
User location | region | A 2-letter code (ISO-3166) that identifies the state, province, or region that the IP address maps to. |
User location | zipCode | The ZIP code or postal code that the IP address maps to. This value is only available for IP addresses associated with the US, Puerto Rico and Canada. |
tip
For details on the specific values that the quirks will be populated with, see the Akamai documentation for the device object and the user location object.
Add quirk definitions#
In the previous section you configured the EdgeWorker to make quirks available to the classification process. In order for personalization rules to be configured to use the quirks, the quirks must be defined in Uniform.
Pre-configured quirk definitions#
If you want to personalize using any of the following quirks, you should install the Akamai integration:
Category | Configuration name | Public ID | Definition name |
---|---|---|---|
Device | brandName | aka-device-brandName | Device Brand Name |
Device | isMobile | aka-device-isMobile | Device Is Mobile |
Device | isTablet | aka-device-isTablet | Device Is Tablet |
Device | modelName | aka-device-modelName | Device Model Name |
Device | os | aka-device-os | Device Operating System |
User location | city | aka-loc-city | City |
User location | continent | aka-loc-continent | Continent Code |
User location | country | aka-loc-country | Country Code |
User location | region | aka-loc-region | Region Code |
In Uniform, open your project.
Navigate to Settings > Integrations.
Click Akamai.
Click Add to project.
Navigate to Optimization > Quirks.
About this step
You will see a list of quirks that were added by the Akamai integration.
Manual quirk definition#
If you want to personalize using any of the following quirks, you must define the quirks yourself:
Category | Configuration name | Public ID | Data type |
---|---|---|---|
Device | acceptsThirdPartyCookie | aka-device-acceptsThirdPartyCookie | List: true/false |
Device | hasAjaxSupport | aka-device-hasAjaxSupport | List: true/false |
Device | hasCookieSupport | aka-device-hasCookieSupport | List: true/false |
Device | hasFlashSupport | aka-device-hasFlashSupport | List: true/false |
Device | isWireless | aka-device-isWireless | List: true/false |
Device | marketingName | aka-device-marketingName | Text |
Device | mobileBrowser | aka-device-mobileBrowser | Text |
Device | mobileBrowserVersion | aka-device-mobileBrowserVersion | Text |
Device | osVersion | aka-device-osVersion | Text |
Device | physicalScreenHeight | aka-device-physicalScreenHeight | Text |
Device | physicalScreenWidth | aka-device-physicalScreenWidth | Text |
Device | resolutionHeight | aka-device-resolutionHeight | Text |
Device | resolutionWidth | aka-device-resolutionWidth | Text |
Device | xhtmlSupportLevel | aka-device-xhtmlSupportLevel | Text |
User location | zipCode | aka-loc-zipCode | Text |
About this step
When you configure these quirks, you must use the public ID from the table above. This is because the logic in the EdgeWorker will set the quirk values using these values.
Create signal using quirk#
Now you can create signals using the quirks you added.
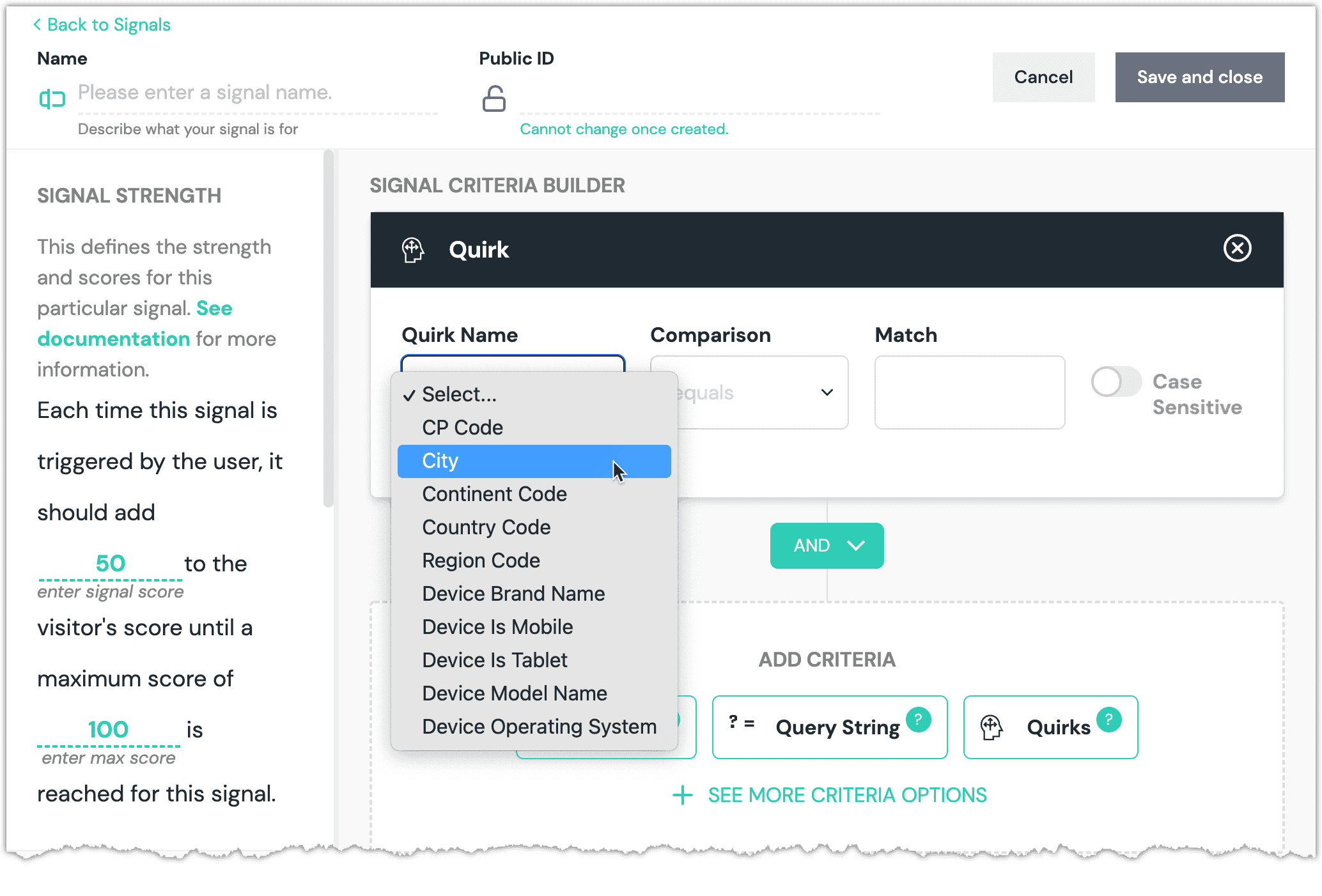
tip
All the defined quirks are listed here, even if the quirk was not specified in the EdgeWorker. If you select a quirk that's not populated, you won't get an error; the quirk won't exist (i.e. the signal operator exists
will be false
and the operator does not exist
will be true
. ).