Activate inline editing
Inline editing makes content editable within your compositions directly inside the preview section of the Canvas editor. This is similar to the way you may expect to interact with content within text processors like Microsoft Word or Notion, or a design tools such as Figma or Canva. It supports real-time editing of simple and multi-line text fields.
This feature helps content authors edit seamlessly, without needing to know which parameter contains what content. Once configured, you'll see a gray, dashed outline around the active element. This is your indication that an element is editable.
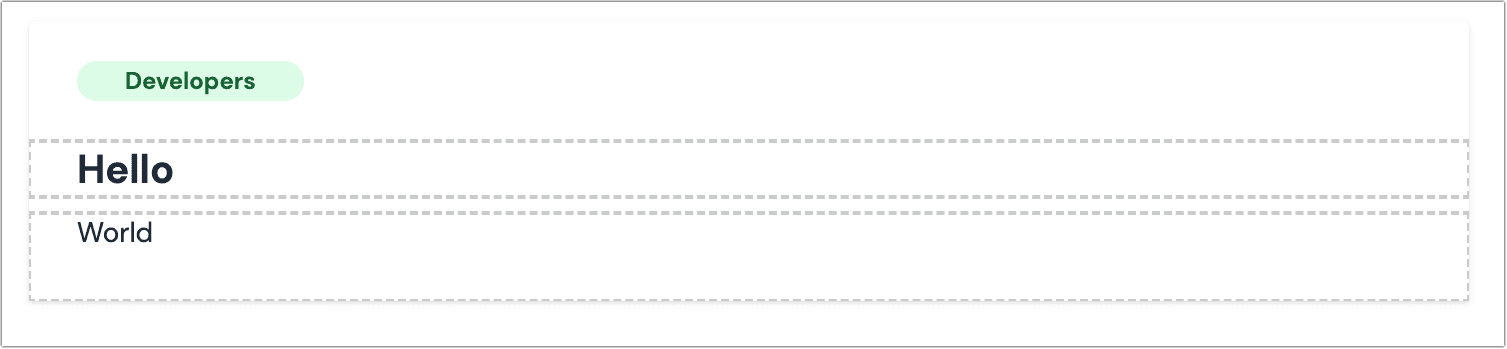
If you see some text within Canvas that's not editable (such as, there is no gray, dashed outline) it's likely because:
- The text isn't managed within Uniform (for example, it's static text from your front-end application)
- The text isn't coming from a parameter of type
text
(it might be coming for another parameter type, such as a CMS entry). - The parameter isn't editable (you don’t have permissions, it’s part of a pattern, or it’s connected to a data type).
- Your frontend team isn't using
<UniformText />
to render the parameter. - The text is coming from a rich text parameter type.
Configure inline editing#
attention
Inline editing requires visual editing to be activated first.
To enable editing text directly in the preview panel, you need to use <UniformText />
in your frontend app.
This component renders an HTML element with some attributes which tells Uniform to enable inline editing for the parameters.
Usage#
info
<UniformText />
must be a descendant of a Uniform component (not necessarily a direct child). See the Examples section for full component examples.
Supported props#
Prop name | Type | Description |
---|---|---|
parameterId | string Required | This is the public id of the parameter. This value can be found in the definition of the component. |
as | string Defaults to span | The HTML element to render. |
isMultiline | boolean Defaults to false | Used to indicate that the parameter supports multi-line. When set to true, <UniformText/> will add the CSS property white-space: 'pre-wrap' which should allow rendering line breaks without the need for <br /> . |
render | Function Defaults to (value) => value | This function allows the developers to customize a parameter value that supporting inline editing. This can be useful when you want to format the parameter before rendering it. Use cases include:
|
placeholder | string | (param: {id: string}) => string Defaults to null | The value to show in Canvas editor when the parameter value is empty. This property is used only in Contextual Editing mode inside Canvas editor, and doesn’t affect the actual value of the parameter. |
Examples#
Let’s imagine a hero component with a parameter title with the public ID title
.
Simple#
Custom HTML element#
Custom rendering#
With placeholders#
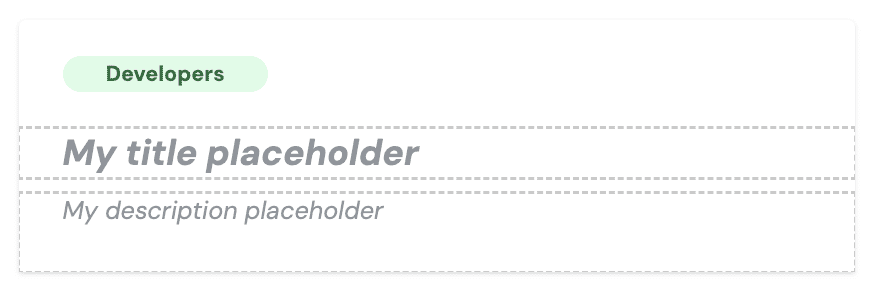
Using default placeholder#
You can also set a default placeholder on the composition level:
getParameterAttributes#
We also provide a helper to annotate the parameters without using <UniformText />
, for when your app is using React Server Components or similar.
Constraints#
- This feature doesn't allow access to text from an external CMS. Its intent is to facilitate the editing of Uniform content.
- Uniform provides some help functions for React Server Components which will return attributes you can attach to your elements. This is a standard approach.
- The logic inside
<UniformText/>
is kept to a minimum, with the majority work done by a script injected into preview mode. This isn't part of your application's bundle, keeping size and JavaScript additions minimal.