Activate visual editing
Uniform Canvas offers a visual editing workspace, which facilitates making structural changes directly from within the preview panel. This is available for both compositions and patterns.
Uniform provides SDKs for visual editing for Next.js and Nuxt 3.
Enable preview support in code#
Prerequisite
These instructions assume you already have enabled rendering of Canvas compositions in your front-end application. For instructions, see the rendering section.
How you activate visual editing in your application depends on the technology used to build the front end.
Troubles enabling preview?
Consult the troubleshooting guide if you have any issues with enabling preview mode.
Next.js#
info
These instructions apply to Next.js projects using the Pages Router. If you're using the App Router instead, please refer to our Next.js App Router tutorial.
Add preview route handler#
After you have activated composition rendering in your front-end application, you need to add a preview route.
A number of new files are needed to support visual editing.
Start your front-end application
Add the following values to your
.env
file:Environment variable Description UNIFORM_PREVIEW_SECRET
This can be any value you want. However, this value must be included in the preview URL that you will configure in a later step. About this step
Technically you don't need to set the preview secret in an environment variable. You could hard-code the value into your app, but using environment variables is a "best practice."
Install dependencies
npm install @uniformdev/canvas-nextAdd the following preview handler:
import { createPreviewHandler } from '@uniformdev/canvas-next' const handler = createPreviewHandler({ secret: () => process.env.UNIFORM_PREVIEW_SECRET, }) export default handlerAbout this step
This adds a handler to redirect preview requests to the right page. You can read more about Preview Mode in Next.js docs.
Customize preview route handler#
The previous code snippet works for simple projects that have basic routing and don't need enhancers. For more advanced projects you can customize the preview route handler to support enhancers and custom routing.
Enabling enhancers in preview mode#
If your project uses enhancers, the following changes need to be added to the preview route handler to ensure that the enhancers are executed in preview mode when the composition is updated in Canvas.
Custom routing in preview mode#
In some cases you might want to have a different URL for your composition previews. By specifying a custom resolveFullPath
function you can construct your own URL that will be used for the preview mode.
Nuxt 3#
The Uniform module for Nuxt integrates preview mode out of the box. No front-end application modifications are needed. But you still need to configure the Uniform project in order for the preview button to work.
pages/[...slug].vue
Inline editing#
Inline editing allows content editors to make changes to the text of a component directly in the preview panel.
Refer to the inline editing guide to learn how to enable inline editing in your components.
Placeholders for empty slots#
When a composition has an empty slot, you'll see a placeholder as shown in the screenshot below:
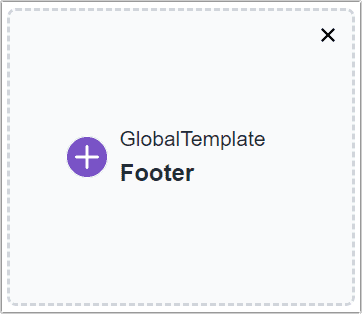
These placeholders help content editors be aware of the slot's existence and have a visual way to add a component to the empty slot directly in the preview panel. Each placeholder can be dismissed by clicking on the X icon. This preference is saved in the browser's local storage, so it will not be shown again for that slot on the same composition.
Customize placeholders#
To match the dimensions of a real components, the placeholder is based on one of the allowed components in that specific slot.
But it might happen that the placeholder doesn't look exactly as expected. In this case you can control the dimensions of the placeholder by rendering a custom component.
Disable placeholders#
If you don't want to show placeholders for a specific slot of a component then you can disable them:
Visual editing for compositions#
warning
Visual editing requires preview mode be activated first.
Visual editing is enabled by default when using <UniformComposition />
to render your compositions. See the Next.js with enhancers tab for requirements for that use case.
Visual editing is enabled by default when using <UniformComposition />
to render your compositions. If you're using useUniformComposition
, the enhancer will be automatically inherited by <UniformComposition />
. Otherwise, you'll need to pass it manually via the contextualEditingEnhancer
prop.
Visual editing is enabled by default when using <UniformComposition />
to render your compositions. If you're using enhancers, make sure you pass the enhancing function to the contextualEditingEnhancer
prop.
Visual editing for patterns#
Unlike compositions, component patterns or composition patterns don't have a project map node assigned. This means that the application doesn't know which route in your front-end app can preview a pattern.
To make this possible, you need to build a page dedicated to previewing patterns. You'll create a new route in your front-end application (for example, /playground
), add <UniformPlayground />
to that page, and then let the SDK know where to redirect the pattern preview requests, using the playgroundPath
attribute. See the framework-specific instructions below to learn how to achieve that.
Create a new route for the preview playground, for example:
pages/playground.tsx
orapp/playground/page.tsx
.Add
<UniformPlayground />
to the page.import { UniformPlayground } from '@uniformdev/canvas-react'; import '../components'; export default function Page() { return <UniformPlayground /> }info
../components
is used as an example here, but you need to import a file that registers all the possible components.In
/api/preview.tsx
add theplaygroundPath
attribute tocreatePreviewHandler
.import { createPreviewHandler } from '@uniformdev/canvas-next'; const handler = createPreviewHandler({ playgroundPath: '/playground', // ...otherAttributes }); export default handler;
Decorators#
warning
This is an experimental feature. Subject to breaking changes in future releases.
Patterns are designed for use inside other compositions, but an isolated preview of the pattern might not give an accurate representation of how the pattern will look inside a composition. To improve this, Uniform has introduced a concept of "decorators," which wrap patterns with additional rendering functionality.
Decorators are typically used to stand in for the composition where the pattern will be used, or to add some rendering controls (re-sizer, background color changer, etc.).
To create a decorator, you can use the type UniformPlaygroundDecorator for guidance. The decorator component receives 2 props:
children
(ReactNode) the rendered pattern, with any prior decorators applied to it.data
(RootComponentInstance) the data of the pattern. For instance, this can be used to render a specific mock per component type.
Decorator definition:
Decorator usage:
Examples
Resize the container
tip
To allow interacting with dynamic decorators even when Page Interactions are disabled, you need to add IS_RENDERED_BY_UNIFORM_ATTRIBUTE
to the clickable elements.
Create a new route for the preview playground, for example:
pages/playground.vue
.Add <UniformPlayground /> to the page.
<script setup> import { resolveRenderer } from '../components'; </script> <template> <UniformPlayground :resolve-renderer="resolveRenderer" /> </template>info
resolveRenderer
is a function that should return the Vue components to render for each Uniform component. It should include all the possible components.In
nuxt.config.ts
add theplaygroundPath
attribute to Uniform module's config.export default defineNuxtConfig({ uniform: { playgroundPath: '/playground', // ...otherAttributes }, });
You can use custom enhancers within the playground by implementing the following code:
../components
is used as an example here, but you need to import a file that registers all the possible components.