Rich text support
Rich text gives editors control over how text and other elements appear within a component. This could be as simple as allowing inline formatting in the form of bold and italic text effects, or more specialized elements such as block quotes, code blocks, or even images or tables.
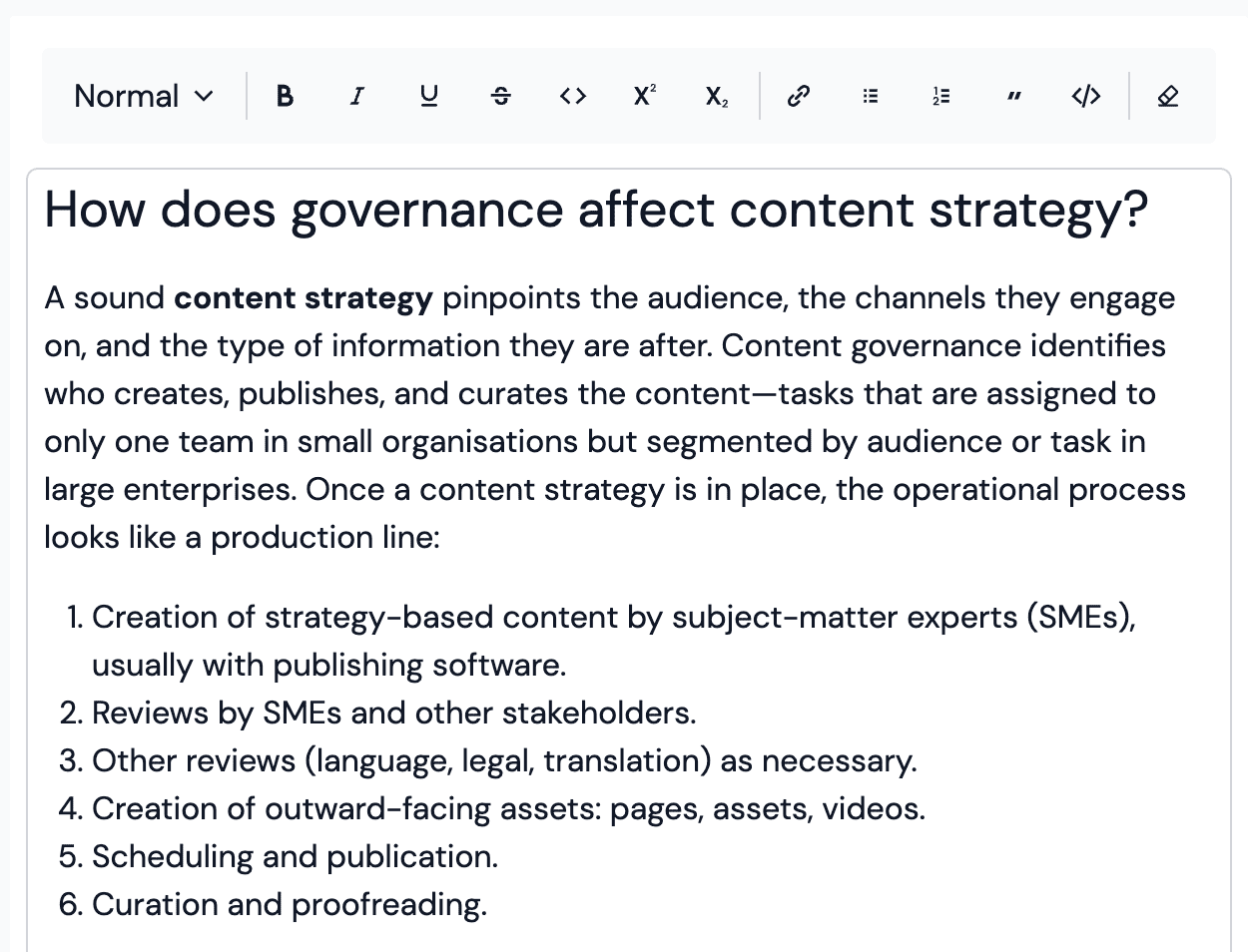
Rich text in Uniform#
Most systems distill the formatting from rich text into a proprietary JSON format that's programmatically consumed by your chosen front end, converting the raw data into web-friendly HTML.
Rich text fields can vary dramatically in terms of their function and capabilities. Most systems supporting this formatting will also provide a proprietary package or rendering engine that does the heavy lifting so that rich text works seamlessly when computed into HTML.
Rich text from Uniform or external sources#
An important early decision to make is if you want your editors to create and maintain rich text for a given component within Uniform directly or from an external system. Unlike many parameters in Uniform, the Rich Text parameter isn't designed to be connected to external data as Uniform can't guarantee that external sources utilise a compatible data structure to the one supported by Uniform's packages.
Uniform's Rich Text parameter#
Out of the box you will find that Uniform supplies a parameter type called Rich Text that can be implemented on any component or content type. Once implemented, the parameter will allow you to enter and format rich text directly in compositions and entries.
Add a Rich Text parameter to a component#
Adding the Uniform Rich Text parameter is the same as adding any other parameter type. Navigate to the component definition which you would like to add a Rich Text property to and select Add parameter. Then select the Rich Text parameter type:
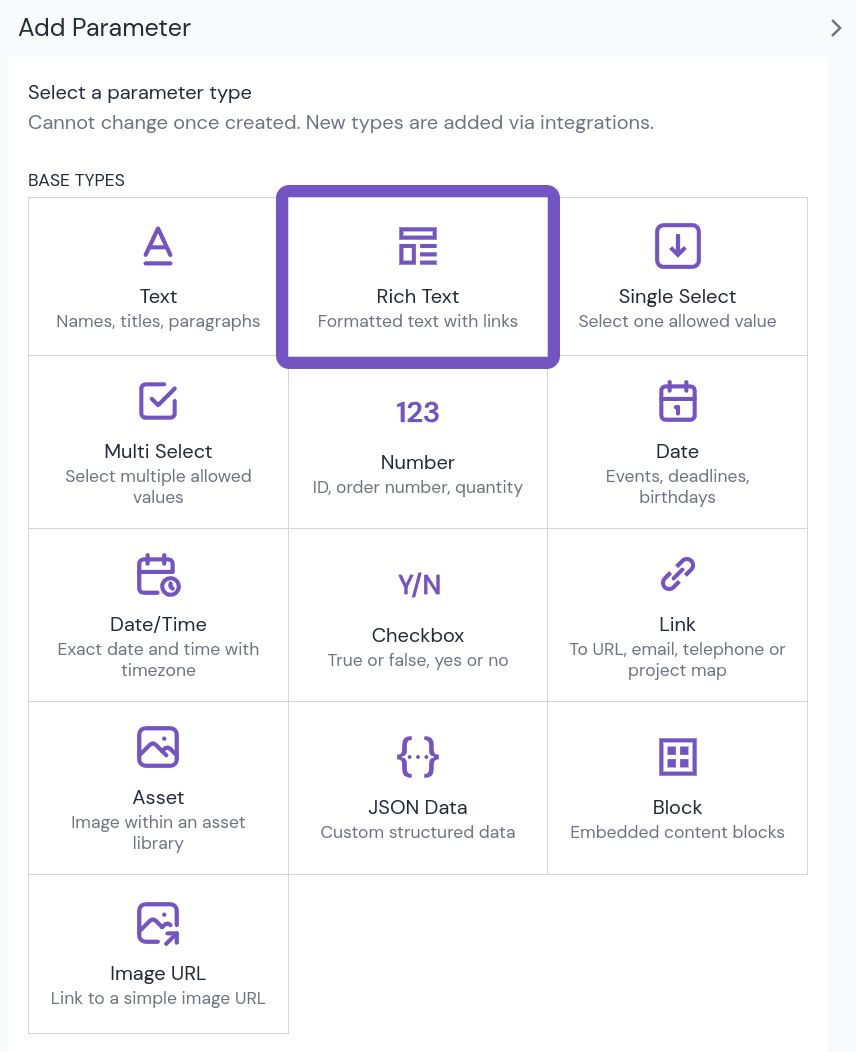
Once added, you will be able to control the formatting options that you would like to make available to your editors. This provides necessary governance to prevent authors from using elements that aren't part of the design system (such as block quotes).
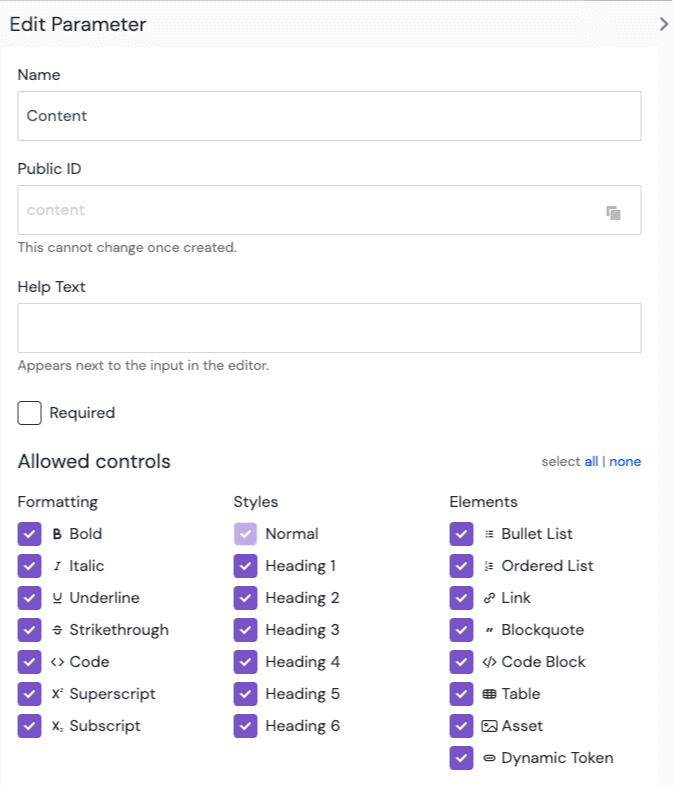
Once your new parameter has been saved, configuration is complete. Next, you will need to ensure that your front end is able to process the rich text field, converting it to web-friendly HTML.
Render the Uniform Rich Text parameter#
Uniform stores any content that's entered into the Rich Text parameter in a JSON format delivered through Uniform's APIs and compiled into HTML. Below are some ways you can perform this compilation of HTML using the Uniform SDK.
Uniform provides a Vanilla.js-based rich text to HTML renderer as well as components for React and Vue. Each option allows for overriding the way a rich text node is rendered.
There are two types of React or Vue components:
UniformRichText
which is given aparameterId
. It will look up the value based on a current component. This is likely to be the simplest way to render rich text fields within the application.Rich Text in Blocks
Rich Text parameters nested within a block parameter are not currently supported by the
UniformRichText
component. Use theUniformRichTextNode
component instead.- The
UniformRichTextNode
component which can be given the node data directly.
Uniform also supplies framework-specific components for Next.js and Nuxt. These take care of overriding some nodes which have framework-specific functionality like the link
node.
Render a Rich Text parameter#
Next.js
If you are using Next.js, import the same component from the @uniformdev/canvas-next
package to get enhancements based on the framework.
Render a rich text node#
Rich text content will look something like this:
Check if the Rich Text parameter is empty#
Compared to simpler parameters, the Rich Text parameter value is a complex JSON object, so checking if it's empty can be more complicated than comparing the value to an empty string or undefined
. To help with this task, the @uniformdev/richtext
package exports an isRichTextValueConsideredEmpty
function that you can use to check if the parameter is empty.
Rendering overrides#
You can override internal rich text node renderers:
Following is the list of internal node types and their default render elements:
Node | node.type | Default renderer | Notes |
---|---|---|---|
Heading | heading | 'h1' | 'h2' | 'h3' | 'h4' | 'h5' | 'h6' | |
Paragraph | paragraph | 'p' | |
List | list | 'ul' | 'ol' | |
List item | listitem | 'li' | |
Link | link | 'a' | |
Asset | asset | 'figure' | Requires version >= 19.187.0 of the Uniform SDKs |
Table | table | 'table > tbody' | Requires version >= 19.181.1 of the Uniform SDKs |
Table Row | tablerow | 'tr' | Requires version >= 19.181.1 of the Uniform SDKs |
Table Cell | tablecell | 'td' | 'th' | Requires version >= 19.181.1 of the Uniform SDKs |
Text | text | <RAW VALUE> | |
Tab | tab | \t |
In addition, some nodes have additional properties on them that can be useful when rendering:
node.type | Properties | Notes |
---|---|---|
heading | tag - 'h1' | 'h2' | 'h3' | 'h4' | 'h5' | 'h6' | |
paragraph |
| Use the |
|
| If |
list-item | value - number | If value is > 0, you should set it to the list item value attribute. |
link | link - { type: 'projectMapNode' | 'url' | 'tel' | 'email'; path: string; nodeId?: string; projectMapId?: string; } | Use the linkParamValueToHref helper exported from Uniform @uniformdev/richtext SDK to convert a link parameter value to a href attribute. |
asset | __asset - Properties of the embedded asset | |
table | ||
tablerow | ||
|
|
Use the |
|
| Use the |
tab |
Rich text from integrations and external sources#
The Uniform Rich Text parameter is ideal for components leveraging a single piece of content to support an article or campaign. But sometimes you may use structured content from multiple external sources. In these cases, JSON-based rich text that comes from a system with a data structure different from the one provided by Uniform requires slightly different steps to ensure the external rich text renders correctly in your frontend.
Connecting external rich text to components#
To consume rich text data in the frontend, you will need to create a parameter to connect to rich text data in the API response from your chosen external source. First, navigate to the component which you would like to support rich text, and add a new parameter of type JSON Data. Give this parameter any name that you like, for example "Rich text content."
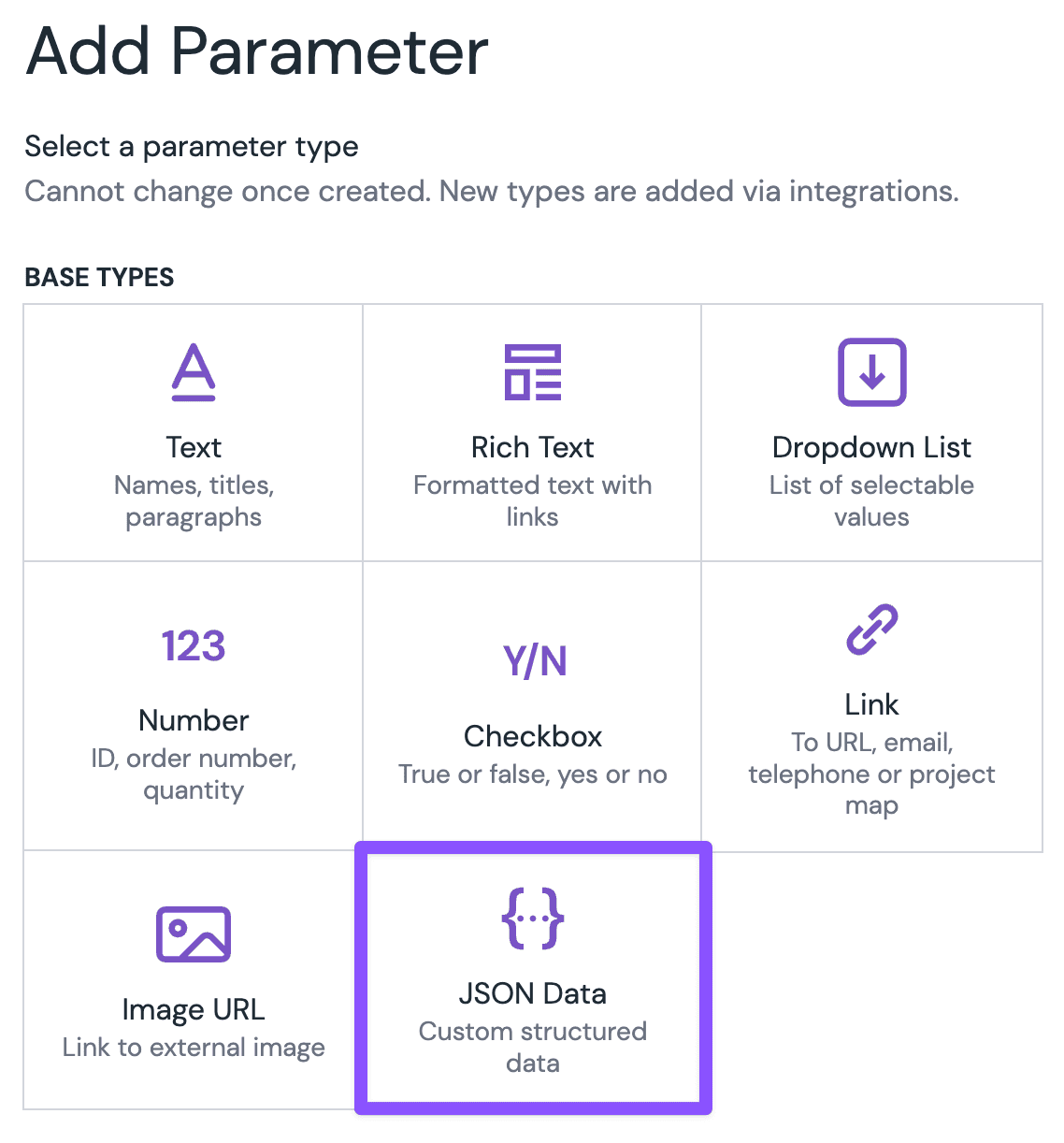
Once the parameter is configured, you can connect it to any JSON content from external sources. Next, within Canvas, you will create a new data resource which contains rich text content from your integration source. At this stage, you need to select the top-level field which contains the rich text content in JSON format, and connect this data to your component.
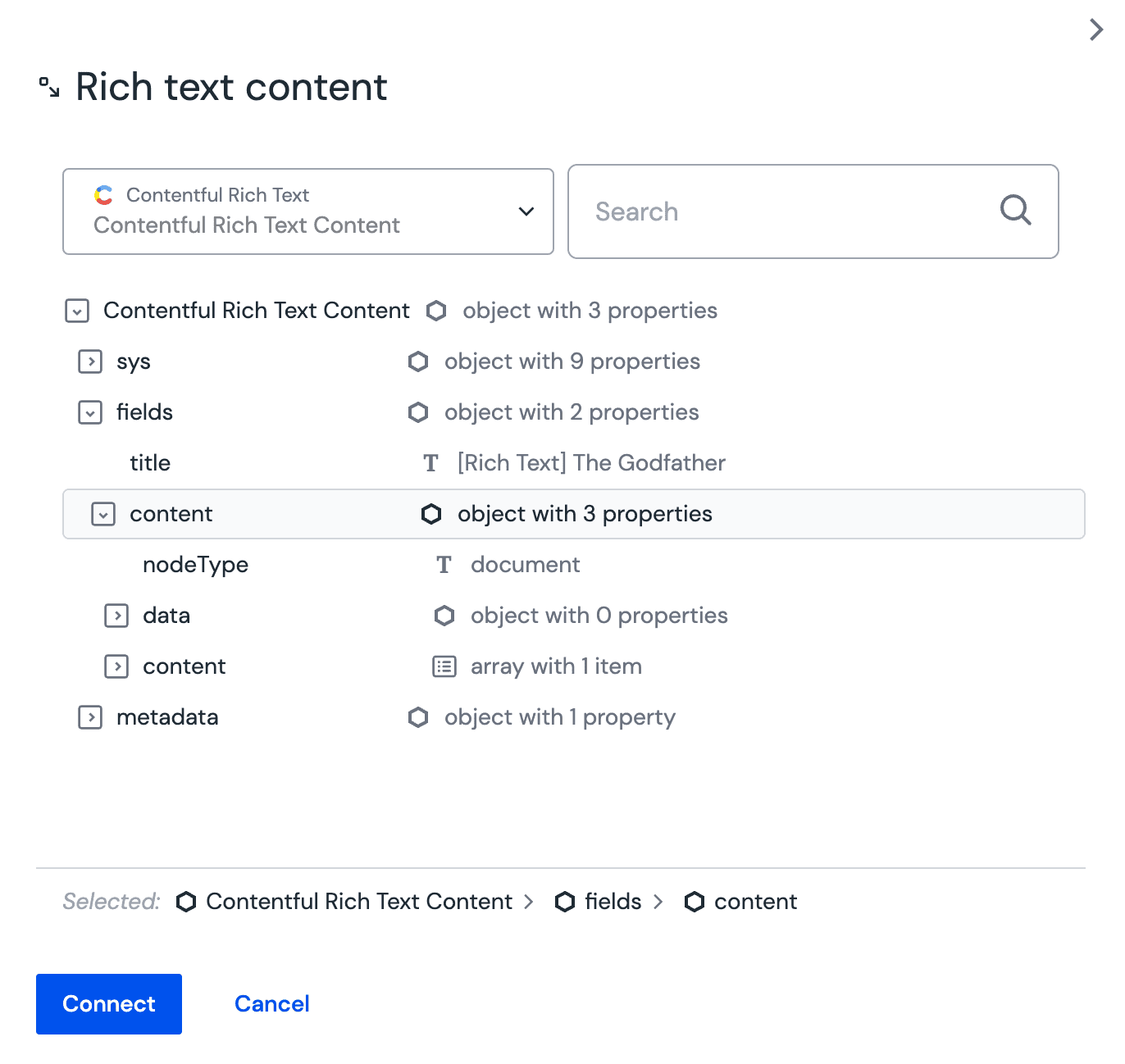
tip
Once you have set up a data connection between a JSON parameter and rich text, Uniform recommends saving this configuration as a pattern to make updating the content easier in the future. To find out more, check out the guide on patterns.
To learn more about connecting data to components, check out the connect external data guide.
Rendering external rich text into HTML#
Once you have connected the JSON rich text data to your component in Canvas, you will need to head to the component on your front end which will resolve the rich text into HTML. Check the documentation for your rich text service, as the steps for processing rich text may vary by front-end framework.
In general, this may involve installing a package from the service that can handle the compilation of HTML from the JSON data that you have already connected to Canvas.
Connecting multiple rich text sources
If you are using a project with multiple integrations, there may be a case where you need to support rich text from multiple sources on the same component. In these cases, Uniform recommends following the same steps as above and to use the frontend to determine the correct package for compiling the resulting JSON data into HTML.