BigCommerce parameters
After you install the BigCommerce integration, the following parameter types are available. You can use these to incorporate content from BigCommerce into your components and compositions.
Parameter type | Description |
---|---|
BigCommerce Product | Select a single product from BigCommerce. |
BigCommerce Product List | Select multiple products from BigCommerce. |
BigCommerce Product Query | Specify search criteria that's used to select a single or multiple products from BigCommerce. |
BigCommerce product#
This parameter type allows a Uniform user to select a single BigCommerce product.
Add parameter to component#
To allow a user to select a product from BigCommerce, you must add a parameter to a component. The parameter is used to store the identifier to the selected product when the user selects a product.
In Uniform, navigate to your component.
Add a new parameter using the parameter type BigCommerce Product.
The following values can be specified:
Name Description Required Indicates whether the value is required when the component is used. BigCommerce Product parameter configuration.
Edit parameter value#
When you use a component with a BigCommerce Product parameter, by default no product will be selected. You are prompted to select a product.
Click Select.
Click the product you want to select.
About this step
A couple of filters are available. The dropdown allows you to filter by content type. The text box allows you to filter by product name.
Click Accept to save your selection.
You will see details about the product you selected, including the title and some metadata.
About this step
After your selection is saved, you are presented with the following options:
- If you want to edit the selected product in BigCommerce, click Edit.
- If you want to deselect the product, click Unlink.
Configure an enhancer#
When a product is selected, Uniform only stores the identifier for the product. Your front-end application must retrieve the details for the product. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with BigCommerce.
How Uniform stores the selected product#
The following is an example of what Uniform stores for the parameter.
The product ID is stored as the value.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to BigCommerce to retrieve data for products.
Add the following npm packages to your front-end application:
@uniformdev/canvas @uniformdev/canvas-bigcommerceIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createBigCommerceEnhancer, createBigCommerceClient, CANVAS_BIGCOMMERCE_PARAMETER_TYPES, } from '@uniformdev/canvas-bigcommerce';Add the following code:
const bigCommerceEnhancer = createBigCommerceEnhancer({ client: createBigCommerceClient({ storeHash: "!!! YOUR BIGCOMMERCE STORE HASH !!!", token: "!!! YOUR BIGCOMMERCE API TOKEN !!!", }), createProductOptions: () => { return { // Modify or exclude this property if you want // more fields returned from the BigCommerce API include_fields: ['id', 'name'], }; }, createProductQueryOptions: () => { return { // Modify or exclude this property if you want // more fields returned from the BigCommerce API include_fields: ['id', 'name'], }; }, });About this step
We recommend you moving the BigCommerce credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
About this step
This registers the enhancer to be used for any occurrence of the BigCommerce Product parameter.
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected BigCommerce product (instead of just being identifiers).
BigCommerce Product List#
This parameter type allows a Uniform user to select multiple BigCommerce products.
Add parameter to component#
To allow a user to select multiple products from BigCommerce, you must add a parameter to a component. The parameter is used to store the identifiers to the selected products when the user selects products.
In Uniform, navigate to your component.
Add a new parameter using the parameter type BigCommerce Product List.
The following values can be specified:
Name Description Required Indicates whether the value is required when the component is used. BigCommerce Product List parameter configuration.
Edit parameter value#
This parameter type works the same way as BigCommerce Product, adding the ability to select multiple products from the list when the parameter value is edited.
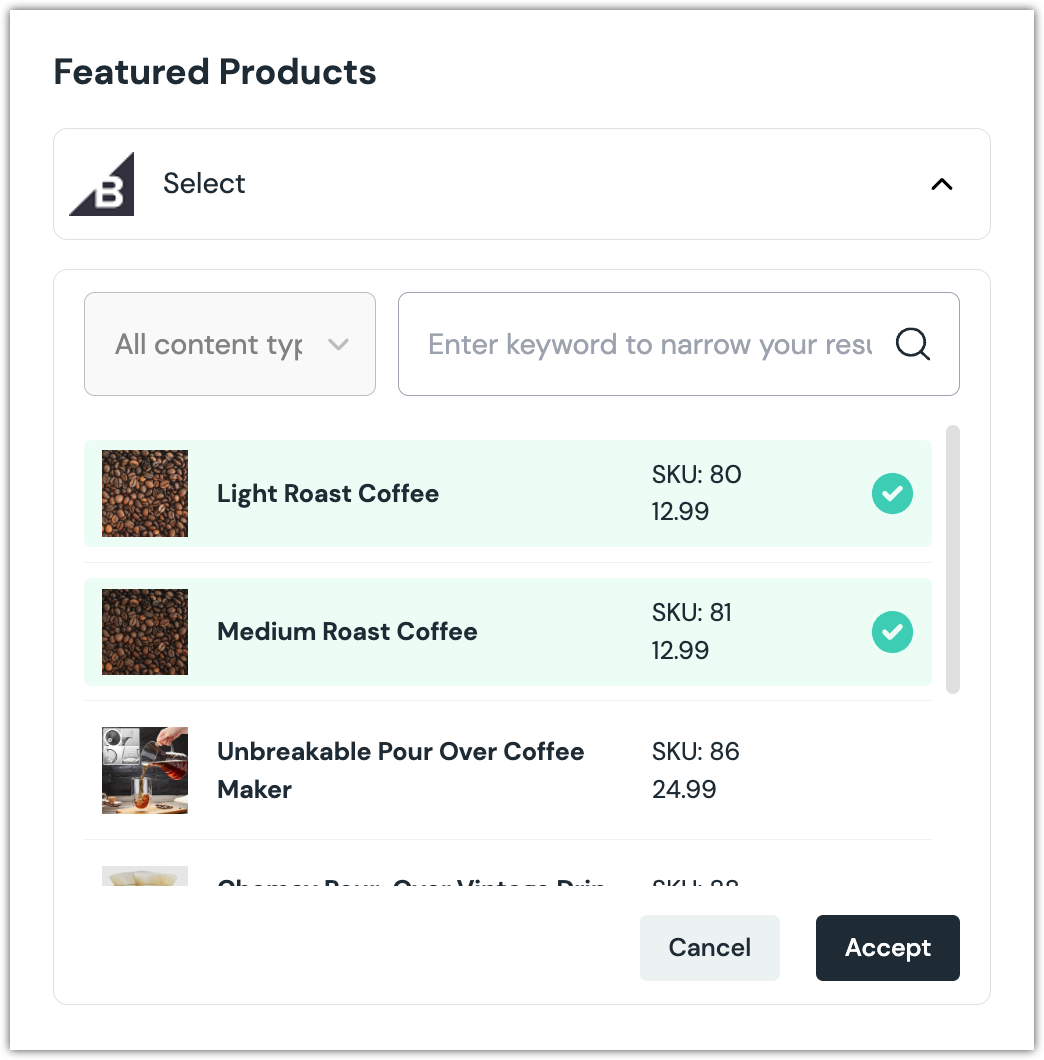
Configure an enhancer#
This parameter type uses the same enhancer as the BigCommerce Product parameter type.
note
The parameter settings are stored in Canvas in a slightly different format. Instead of a single product ID being stored, the value is stored as an array of product IDs.
BigCommerce Product Query#
This parameter type allows a Uniform user to configure a query that will determine the BigCommerce products that are retrieved.
Add parameter to component#
In order to allow a user to configure a query to determine the products from BigCommerce to retrieve, you must add a parameter to a component. The parameter is used to store the query settings used to perform the search.
In Uniform, navigate to your component.
Add a new parameter using the parameter type BigCommerce Product Query.
The following values can be specified:
Name Description Required Indicates whether the value is required when the component is used. BigCommerce Product Query parameter configuration.
Edit parameter value#
Configuring this parameter type involves specifying the way a product search should work. You are able to control the following search settings:
Field | Description |
---|---|
Brand | Only products associated with the selected brand are included. |
Count | The maximum number of products that are returned. |
Keyword | Only products whose names match the specified keyword(s) are included. |
Sort | The product property used to sort the products that are returned. |
Sort Order | Whether the products should be sorted in ascending or descending order. |
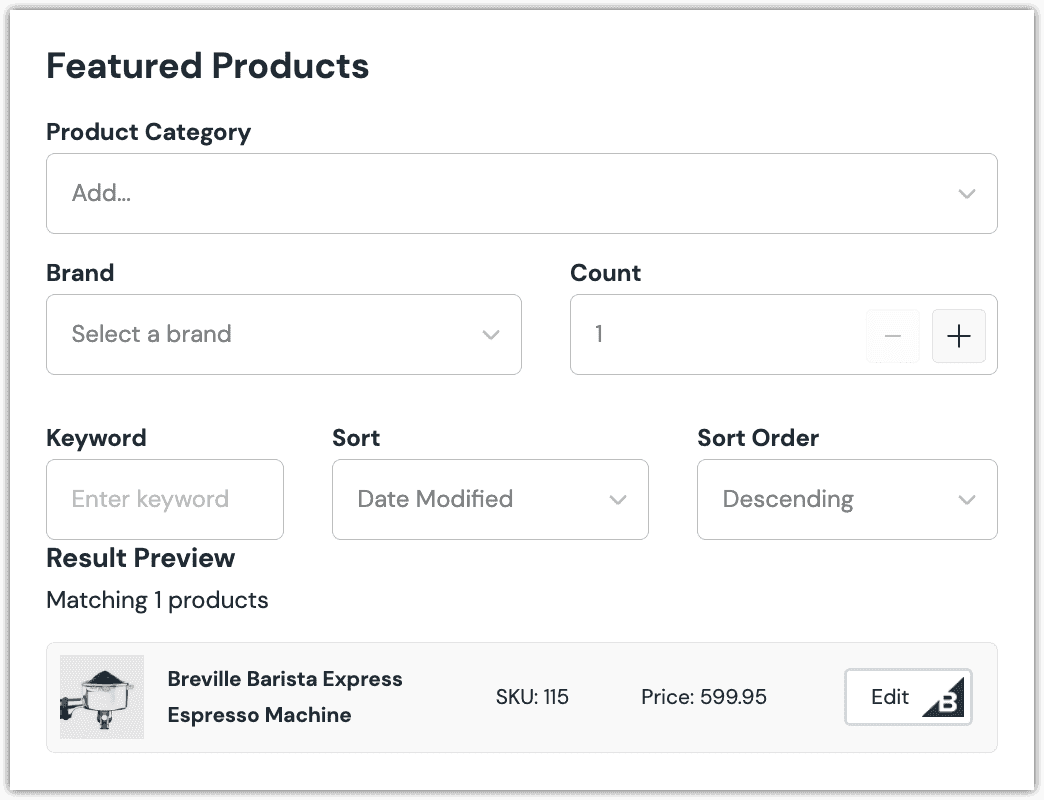
Configure an enhancer#
This parameter type uses the same enhancer as the BigCommerce Product parameter type.
note
The parameter settings are stored in Canvas in a slightly different format. Instead of a single product ID being stored, the value stores the settings used to perform the search.