commercetools parameters
Installing the commercetools integration will cover most use cases without the need for additional code. However, if you need to do any post-processing of any data, or have other specific needs for manipulating data, you can leverage the following parameter types with enhancers.
Parameter type | Description |
---|---|
commercetools Product | Select a single product from commercetools. |
commercetools Product List | Select multiple products from commercetools. |
commercetools Product Query | Specify search criteria that's used to select a single or multiple products from commercetools. |
commercetools Category | Select a specific product category. |
commercetools Product#
This parameter type allows a Uniform user to select a single commercetools product.
Add parameter to component#
To allow a user to select a product from commercetools, you must add a parameter to a component. The parameter is used to store the identifier to the selected product when the user selects a product.
In Uniform, navigate to your component.
Add a new parameter using the parameter type commercetools Product.
The following values can be specified:
Name Description Category constraints Select specific categories that are allowed to appear as results in the product query. Note: Parent categories will include all child categories. Display field Used to update which field is used for titles when previewing the results from the query. Restrict search–Master/Variants Allows you to restrict the result to only include the product master, variants, or both. This can help to reduce the resultant JSON payload and improve site performance. Restrict search–Locale Allows you to remove non-configured locales from results, further reducing potential JSON payload size. Restrict search–Currency Allows you to remove non-configured currencies from results, further reducing potential JSON payload size. Required Indicates whether the value is required when the component is used. commercetools Product parameter configuration.
Edit parameter value#
When you use a component with a commercetools Product parameter, by default no product will be selected. You are prompted to select a product.
Click Select.
Click the product you want to select.
About this step
A couple of filters are available. The dropdown allows you to filter by category. The text box allows you to filter by product name.
Click Accept to save your selection.
You will see details about the product you selected, including the name, category, and price.
About this step
After your selection is saved, you are presented with the following options:
- If you want to deselect the product, click the red X.
Configure an enhancer#
When a product is selected, Uniform only stores the identifier for the product. Your front-end application must retrieve the details for the product. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with commercetools.
How Uniform stores the selected product#
The following is an example of what Uniform stores for the parameter.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to commercetools to retrieve data for products.
Add the following npm packages to your front-end application:
@uniformdev/canvas @uniformdev/canvas-commercetoolsAdd the following file:
import getConfig from 'next/config'; import { ApiRoot, createApiBuilderFromCtpClient } from '@commercetools/platform-sdk'; import { createClient } from '@commercetools/sdk-client'; import { createAuthMiddlewareForClientCredentialsFlow } from '@commercetools/sdk-middleware-auth'; import { createHttpMiddleware } from '@commercetools/sdk-middleware-http'; import { createcommercetoolsEnhancer } from '@uniformdev/canvas-commercetools'; import fetch from 'node-fetch'; const commercetoolsAuthUrl = [!!! YOUR CT AUTH URL !!!]; const commercetoolsProjectKey = [!!! YOUR CT PROJECT KEY !!!]; const commercetoolsClientId = [!!! YOUR CT CLIENT ID !!!]; const commercetoolsClientSecret = [!!! YOUR CT CLIENT SECRET !!!]; const commercetoolsApiUrl = [!!! YOUR CT API URL !!!]; const authMiddleware = createAuthMiddlewareForClientCredentialsFlow({ host: commercetoolsAuthUrl, projectKey: commercetoolsProjectKey, credentials: { clientId: commercetoolsClientId, clientSecret: commercetoolsClientSecret, }, }); const httpMiddleware = createHttpMiddleware({ host: commercetoolsApiUrl, fetch, }); const ctpClient = createClient({ middlewares: [authMiddleware, httpMiddleware], }); const apiRoot: ApiRoot = createApiBuilderFromCtpClient(ctpClient); export const commercetoolsEnhancer = createcommercetoolsEnhancer({ apiRoot, projectKey: commercetoolsProjectKey, });About this step
We recommend you moving the commercetools credentials to environment variables rather than hard-coding them in the front-end app.
In a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { CANVAS_COMMERCETOOLS_PARAMETER_TYPES, } from '@uniformdev/canvas-commercetools'; import { commercetoolsEnhancer } from '../lib/commercetoolsEnhancer';Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_COMMERCETOOLS_PARAMETER_TYPES, commercetoolsEnhancer, ), context: {}, });About this step
This registers the enhancer to be used for any occurrence of the commercetools Product parameter.
Next steps
Now, the parameter value in the composition is mutated to include the field values for the selected commercetools product (instead of just being identifiers).
commercetools Product List#
This parameter type allows a Uniform user to select multiple commercetools products.
Add parameter to component#
To allow a user to select multiple products from commercetools, you must add a parameter to a component. The parameter is used to store the identifiers to the selected products when the user selects products.
In Uniform, navigate to your component.
Add a new parameter using the parameter type commercetools Product List.
The following values can be specified:
Name Description Category constraints Select specific categories that are allowed to appear as results in the product query. Note: Parent categories will include all child categories. Display field Used to update which field is used for titles when previewing the results from the query. Restrict search–Master/Variants Allows you to restrict the result to only include the product master, variants, or both. This can help to reduce the resultant JSON payload and improve site performance. Restrict search–Locale Allows you to remove non-configured locales from results, further reducing potential JSON payload size. Restrict search–Currency Allows you to remove non-configured currencies from results, further reducing potential JSON payload size. Required Indicates whether the value is required when the component is used. commercetools Product List parameter configuration.
Edit parameter value#
This parameter type works the same way as commercetools Product, adding the ability to select multiple products from the list when the parameter value is edited.
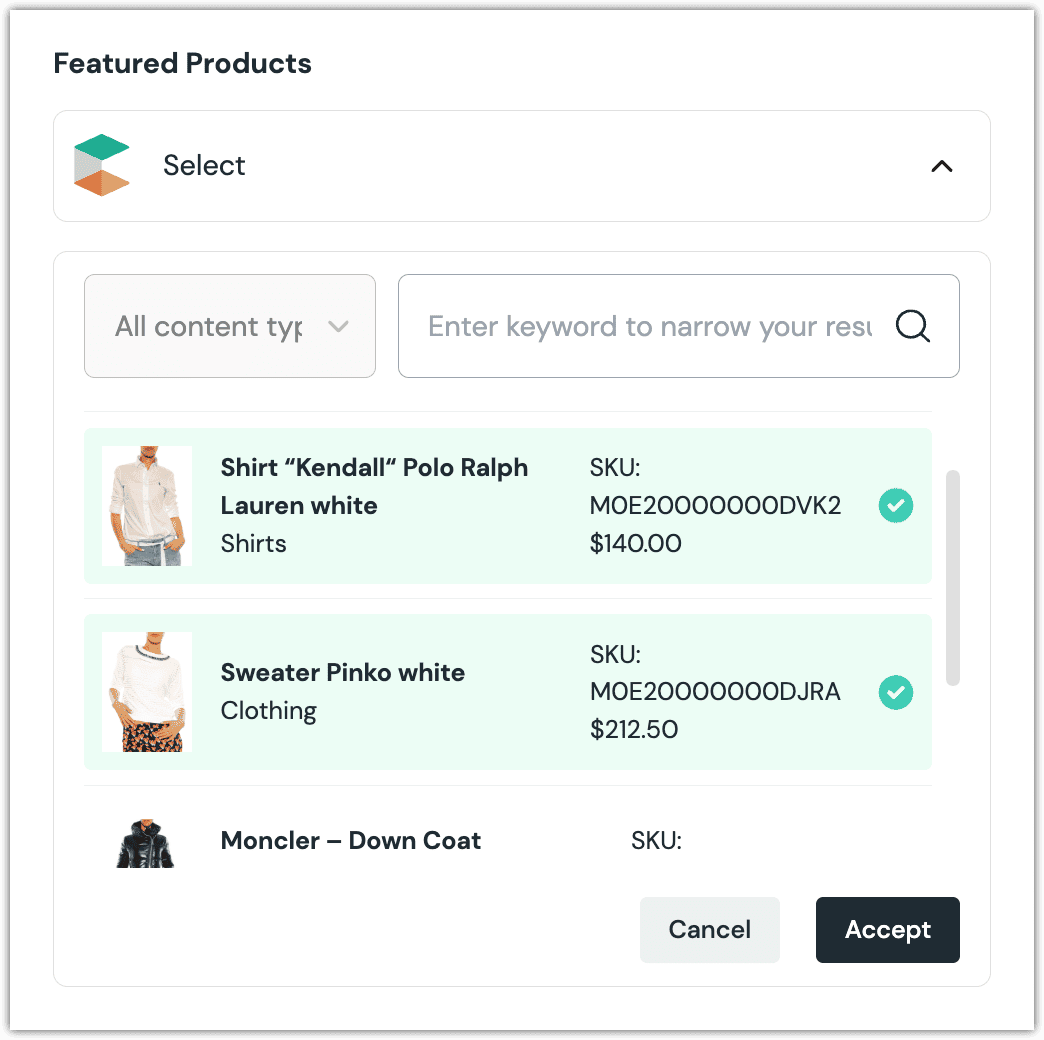
Configure an enhancer#
This parameter type uses the same enhancer as the commercetools Product parameter type.
The following is an example of what Uniform stores for the parameter.
commercetools Product Query#
This parameter type allows a Uniform user to configure a query that will determine which commercetools products are retrieved.
Add parameter to component#
For a user to select categories, you must add the parameter to a component. The parameter is used to store the selected categories from the category selection UI.
In Uniform, navigate to your component.
Add a new parameter using the parameter type commercetools Category.
The following values can be specified:
Name Description Category constraints Select specific categories that are allowed to appear as results in the product query. Note: Parent categories will include all child categories. Display field Used to update which field is used for titles when previewing the results from the query. Restrict search–Master/Variants Allows you to restrict the result to only include the product master, variants, or both. This can help to reduce the resultant JSON payload and improve site performance. Restrict search–Locale Allows you to remove non-configured locales from results, further reducing potential JSON payload size. Restrict search–Currency Allows you to remove non-configured currencies from results, further reducing potential JSON payload size. commercetools Product Query parameter configuration.
Edit parameter value#
Configuring this parameter type involves specifying the way a product search should work. You are able to control the following search settings:
Field | Description |
---|---|
Categories | Filter the query based on the category a product is within. Multiple categories can be selected, and parent categories will include all child categories. |
Count | The maximum number of products that are returned. |
Keyword | Only products whose names match the specified keyword(s) are included. |
Sort | The product property used to sort the products that are returned. |
Sort Order | Whether the products should be sorted in ascending or descending order. |
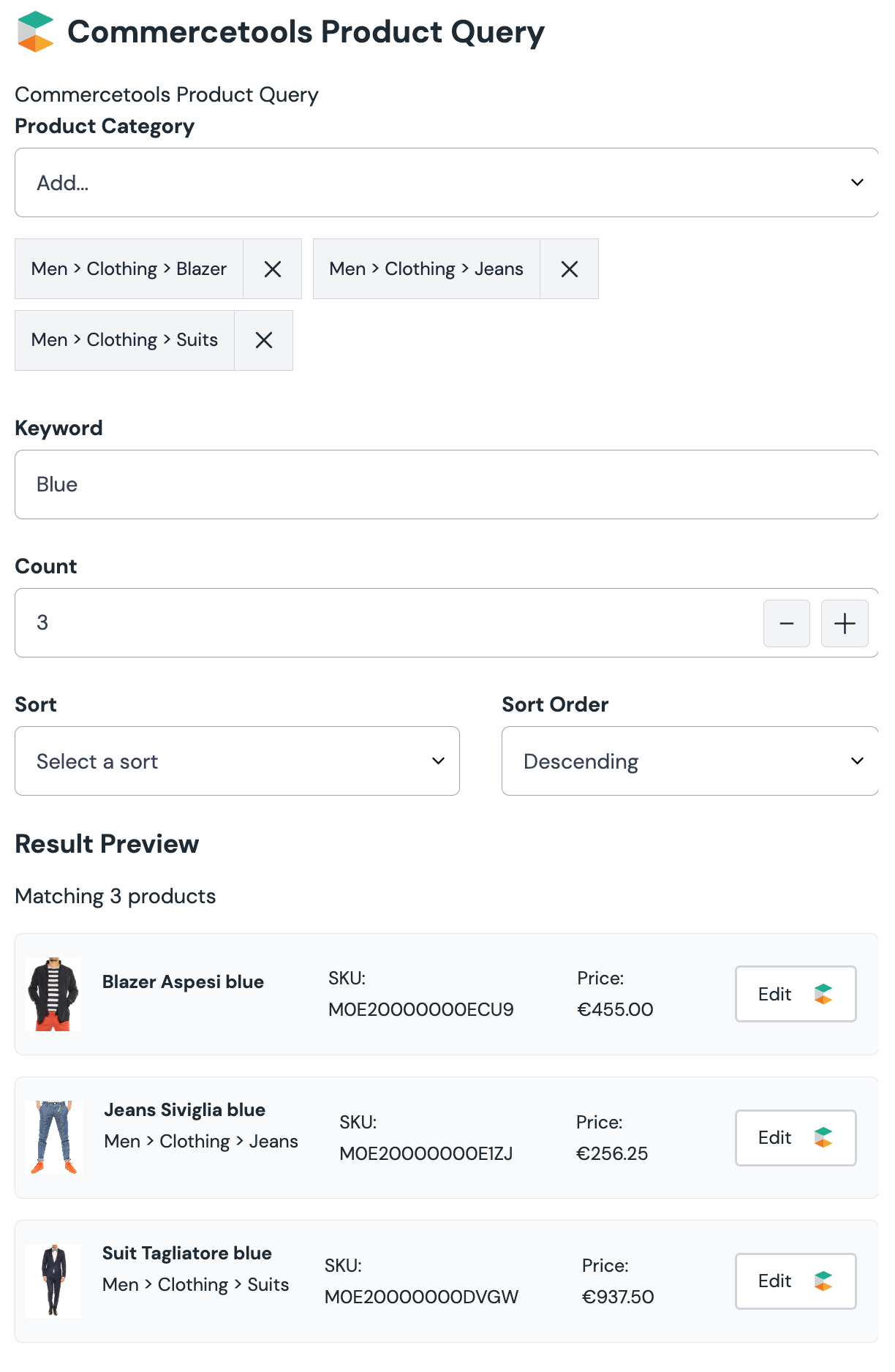
Configure an enhancer#
This parameter type uses the same enhancer as the commercetools Product parameter type.
note
The parameter settings are stored in Canvas in a slightly different format. Instead of a single product ID being stored, the value stores the settings used to perform the search.
commercetools Category#
This parameter type allows a Uniform user to select one or multiple categories to be set as the value of the parameter. This can be useful when composing pages where a global category filter needs to be set, or to access the category description stored in commercetools through the enhancer.
Add parameter to component#
For a user to configure a query to determine the products from commercetools to retrieve, you must add the parameter to a component. The parameter is used to store the query settings used to perform the search.
In Uniform, navigate to your component.
Add a new parameter using the parameter type commercetools Product Query.
The following values can be specified:
Name Description Allow multi select Determines if a user is able to select one or many categories within the category selector. Required Indicates whether the value is required when the component is used. commercetools Category parameter configuration.
Edit parameter value#
When you use a component with a commercetools Category parameter, by default no categories will be selected. You are prompted to select a category.
Click Select.
Click the category or categories you would like to select.
About this step
Sub-categories can be accessed by expanding the parents category with the "+" button on the left-hand side of the category.
Click Save and Close to save your selection.
You will see details about the category or categories you have selected, including the name and slug.
About this step
After your selection is saved, you are presented with the following options:
- If you want to deselect the product, click the Unlink button.
- If you want to add/edit the categories, click the Select Category button.
Configure an enhancer#
This parameter type uses the same enhancer as the commercetools Product parameter type.
note
The parameter settings are stored in Canvas in a slightly different format. Instead of a single product ID being stored, the value stores the settings used to perform the search.