Acoustic parameters
After you install the Acoustic Content integration, the following parameter types are available. You can use these to incorporate content from Acoustic Content into your components and compositions.
Parameter type | Description |
---|---|
Acoustic Content Item | Select a single item from Acoustic Content. |
Acoustic Multi Content Item | Select multiple items from Acoustic Content. |
Acoustic Content Item#
This parameter type allows a Uniform user to select a single Acoustic Content item.
Add parameter to component#
To allow a user to select an item from Acoustic Content, you must add a parameter to a component. The parameter is used to store the identifier to the selected item when the user selects an item.
In Uniform, navigate to your component.
Add a new parameter using the parameter type Acoustic Content Item.
The following values can be specified:
Name Description Select Linked Source Specifies which Acoustic Content subscription is the source for the item that can be selected. Allowed content types Specifies which content types will be available to select from when the parameter value is edited. You can select multiple content types to allow the user to select from a larger set of items. Required Indicates whether the value is required when the component is used. Acoustic Content Item parameter with a single content type selected.
Edit parameter value#
When you use a component with an Acoustic Content Item parameter, by default no items will be selected. You are prompted to select an item.
Click Select.
Click the item you want to select.
About this step
A couple of filters are available. The dropdown allows you to filter by content type. The text box allows you to filter by item content.
Click Accept to save your selection.
You will see details about the item you selected, including the title and some metadata.
About this step
After your selection is saved, you are presented with the following options:
- If you want to edit the selected item in Acoustic Content, click Edit.
- If you want to deselect the item, click Unlink.
Configure an enhancer#
When an item is selected, Uniform only stores the identifier for the item. Your front-end application must retrieve the details for the item. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Acoustic Content.
How Uniform stores the selected item#
The following is an example of what Uniform stores for the parameter.
There are two identifiers in this value:
- The item that was selected.
- The source key for the linked content source.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Acoustic Content to retrieve data for item.
Add the following npm packages to your front-end application:
@uniformdev/canvas-acousticIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { AcousticClientList, CANVAS_ACOUSTIC_CONTENT_ITEM_PARAMETER_TYPES, createAcousticClient, createAcousticContentItemEnhancer, } from '@uniformdev/canvas-acoustic';Add the following code:
const client = createAcousticClient({ apiUrl: '!!! YOUR ACOUSTIC CONTENT API URL !!!', }); const previewClient = createAcousticClient({ apiUrl: '!!! YOUR ACOUSTIC CONTENT PREVIEW API URL !!!', auth: { apiKey: '!!! YOUR ACOUSTIC CONTENT API KEY !!!' }, });About the preview API URL
To determine the appropriate value for the preview API URL, see the preview content section in the Acoustic Content documentation.
About this step
Uniform recommends you moving the Acoustic Content credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
const clients = new AcousticClientList({ client, previewClient }); const acousticEnchancer = createAcousticContentItemEnhancer({ clients });Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_ACOUSTIC_CONTENT_ITEM_PARAMETER_TYPES, acousticEnchancer, ), context: {}, });About this step
This registers the enhancer to be used for any occurrence of the Acoustic Content Item parameter.
Next steps
Now, the parameter value in the composition is mutated to include the field values for the selected Acoustic Content item (instead of just being an identifier).
Acoustic Multi Content Item#
This parameter type is added and edited the same way as Acoustic Content Item. When editing the parameter value, you can select additional items by clicking the button Select new.
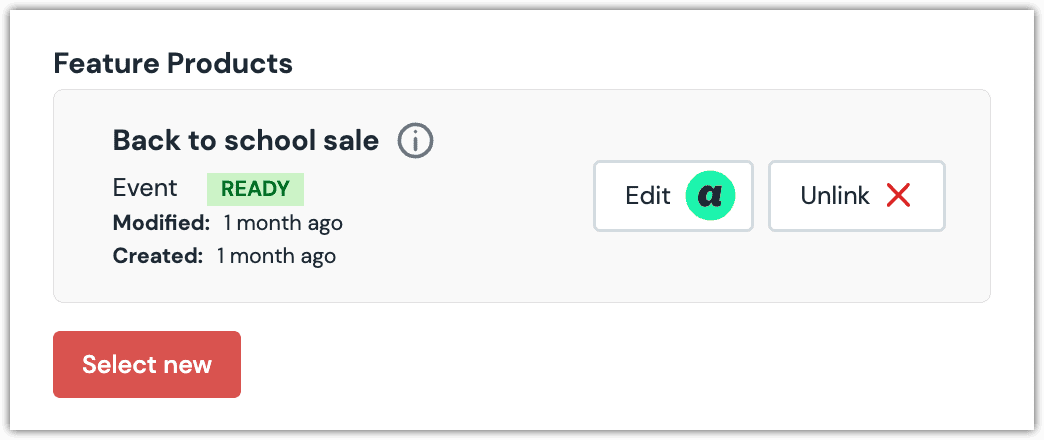
Configure an enhancer#
When items are selected, Uniform only stores the identifiers for the items. Your front-end application must retrieve the details for the items. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Acoustic Content.
How Uniform stores the selected items#
The following is an example of what Uniform stores for the parameter.
There are two identifiers in this value:
- The items that were selected.
- The source key for the linked content source.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Acoustic Content to retrieve data for items.
Add the following npm packages to your front-end application:
@uniformdev/canvas-acousticIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { AcousticClientList, CANVAS_ACOUSTIC_MULTI_CONTENT_ITEM_PARAMETER_TYPES, createAcousticClient, createAcousticContentItemEnhancer, } from '@uniformdev/canvas-acoustic';Add the following code:
const client = createAcousticClient({ apiUrl: '!!! YOUR ACOUSTIC CONTENT API URL !!!', }); const previewClient = createAcousticClient({ apiUrl: '!!! YOUR ACOUSTIC CONTENT PREVIEW API URL !!!', auth: { apiKey: '!!! YOUR ACOUSTIC CONTENT API KEY !!!' }, });
About the preview API URL
To determine the appropriate value for the preview API URL, see the preview content section in the Acoustic Content documentation.
About this step
We recommend you moving the Acoustic Content credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
const clients = new AcousticClientList({ client, previewClient }); const acousticEnchancer = createAcousticContentItemEnhancer({ clients });Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_ACOUSTIC_MULTI_CONTENT_ITEM_PARAMETER_TYPES, acousticEnchancer, ), context: {}, });
About this step
This registers the enhancer to be used for any occurrence of the Acoustic Content Multi Item parameter.
Next steps
Now, the parameter value in the composition is mutated to include the field values for the selected Acoustic Content items (instead of just being identifiers).