Contentful parameters
After you install the Contentful integration, the following parameter types are available. You can use these to incorporate content from Contentful into your components and compositions.
Parameter type | Description |
---|---|
Contentful Entry | Select a single entry from Contentful. |
Contentful Multi Entry | Select multiple entries from Contentful. |
Contentful Query | Specify search criteria that's used to select a single or multiple entries from Contentful. |
Contentful Entry#
This parameter type allows a Uniform user to select a single Contentful entry.
Add parameter to component#
To allow a user to select an entry from Contentful, you must add a parameter to a component. The parameter is used to store the identifier to the selected entry when the user selects an entry.
In Uniform, navigate to your component.
Add a new parameter using the parameter type Contentful Entry.
The following values can be specified:
Name Description Select Linked Source Specifies which Contentful space is the source for the entry that can be selected. Allowed Content Types Specifies which content types will be available to select from when the parameter value is edited. You can select multiple content types in order to allow the user to select from a larger set of entries. Required Indicates whether the value is required when the component is used. Contentful Entry parameter with a single content type selected.
Edit parameter value#
When you use a component with a Contentful Entry parameter, by default no entry will be selected. You are prompted to select an entry.
Click Select.
Click the entry you want to select.
About this step
A couple of filters are available. The dropdown allows you to filter by content type. The text box allows you to filter by entry title.
Click Accept to save your selection.
You will see details about the entry you selected, including the title and some metadata.
About this step
After your selection is saved, you are presented with the following options:
- If you want to edit the selected entry in Contentful, click Edit.
- If you want to deselect the entry, click Unlink.
Configure an enhancer#
When an entry is selected, Uniform only stores the identifier for the entry. Your front-end application must retrieve the details for the entry. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentful.
How Uniform stores the selected entry#
The following is an example of what Uniform stores for the parameter.
There are two identifiers in this value:
- The entry that was selected.
- The source key for the linked content source.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentful to retrieve data for entries.
tip
For more information about the enhancer, see the package documentation.
In Contentful, create a content delivery access token for the enhancer to use to make API calls to Contentful.
Add the following npm packages to your front-end application:
contentful @uniformdev/canvas @uniformdev/canvas-contentfulIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createContentfulEnhancer, ContentfulClientList, CANVAS_CONTENTFUL_PARAMETER_TYPES, } from '@uniformdev/canvas-contentful'; import { createClient } from 'contentful';Add the following code:
const client = createClient({ space: '!!! YOUR CF SPACE ID !!!', environment: '!!! YOUR CF ENVIRONMENT NAME !!!', accessToken: '!!! YOUR CF ACCESS TOKEN !!!', });About this step
We recommend you moving the Contentful credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
const clientList = new ContentfulClientList({ client }); const contentfulEnhancer = createContentfulEnhancer({ client: clientList })Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_CONTENTFUL_PARAMETER_TYPES, contentfulEnhancer ), context: {}, });About this step
This registers the enhancer to be used for any occurrence of the Contentful Entry parameter.
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentful entry (instead of just being identifiers).
Contentful Multi Entry#
This parameter type is added and edited the same way as Contentful Entry. When editing the parameter value, you can select additional entries by clicking the button Select new.
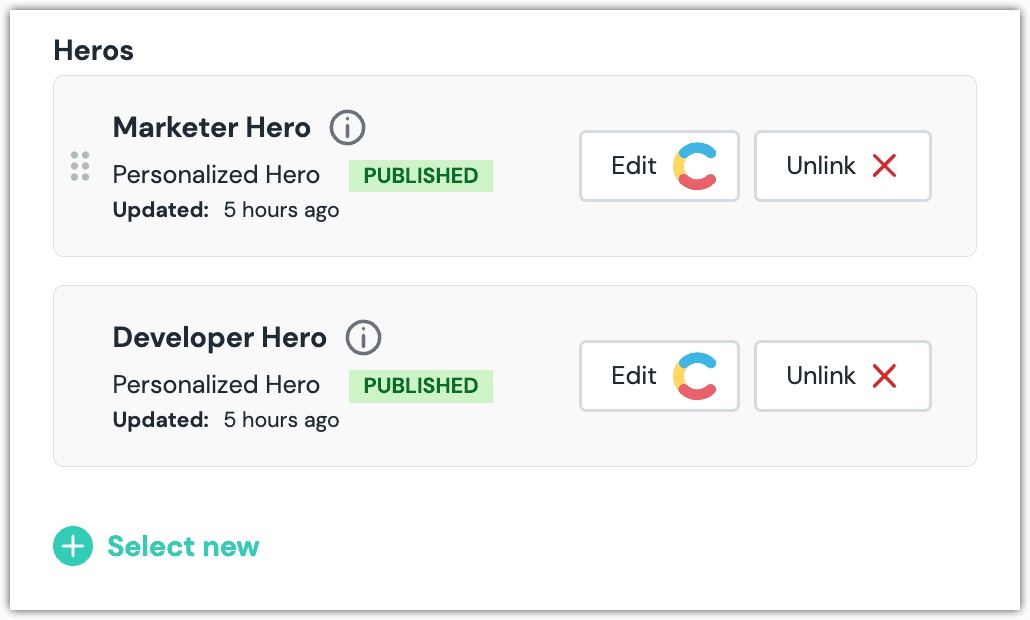
Configure an enhancer#
When entries are selected, Uniform only stores the identifiers for the entries. Your front-end application must retrieve the details for the entries. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentful.
How Uniform stores the selected entries#
The following is an example of what Uniform stores for the parameter.
There are two identifiers in this value:
- An array of the entries that were selected.
- The source key for the linked content source.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentful to retrieve data for entries.
tip
For more information about the enhancer, see the package documentation.
In Contentful, create a content delivery access token for the enhancer to use to make API calls to Contentful.
Add the following npm packages to your front-end application:
contentful @uniformdev/canvas @uniformdev/canvas-contentfulIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createContentfulEnhancer, ContentfulClientList, CANVAS_CONTENTFUL_MULTI_PARAMETER_TYPES, } from '@uniformdev/canvas-contentful'; import { createClient } from 'contentful';Add the following code:
const client = createClient({ space: '!!! YOUR CF SPACE ID !!!', environment: '!!! YOUR CF ENVIRONMENT NAME !!!', accessToken: '!!! YOUR CF ACCESS TOKEN !!!', });About this step
We recommend you moving the Contentful credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
const clientList = new ContentfulClientList({ client }); const contentfulEnhancer = createContentfulEnhancer({ client: clientList })Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_CONTENTFUL_MULTI_PARAMETER_TYPES, contentfulEnhancer ), context: {}, });
About this step
This registers the enhancer to be used for any occurrence of the Contentful Multi Entry parameter.
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentful entry (instead of just being identifiers).
Contentful Query#
This parameter type allows a Uniform user to configure a query that will determine the Contentful entries that are retrieved.
Add parameter to component#
This parameter type is added the same way as Contentful Entry.
Edit parameter value#
Configuring this parameter type involves specifying the way a product search should work. You are able to control the following search settings:
Field | Description |
---|---|
Filter by entry type | Only entries that match the selected entry type are included. |
Count | The maximum number of entries that are returned. |
Sort | The entry field used to sort the entries that are returned. |
Sort Order | Whether the entries should be sorted in ascending or descending order. |
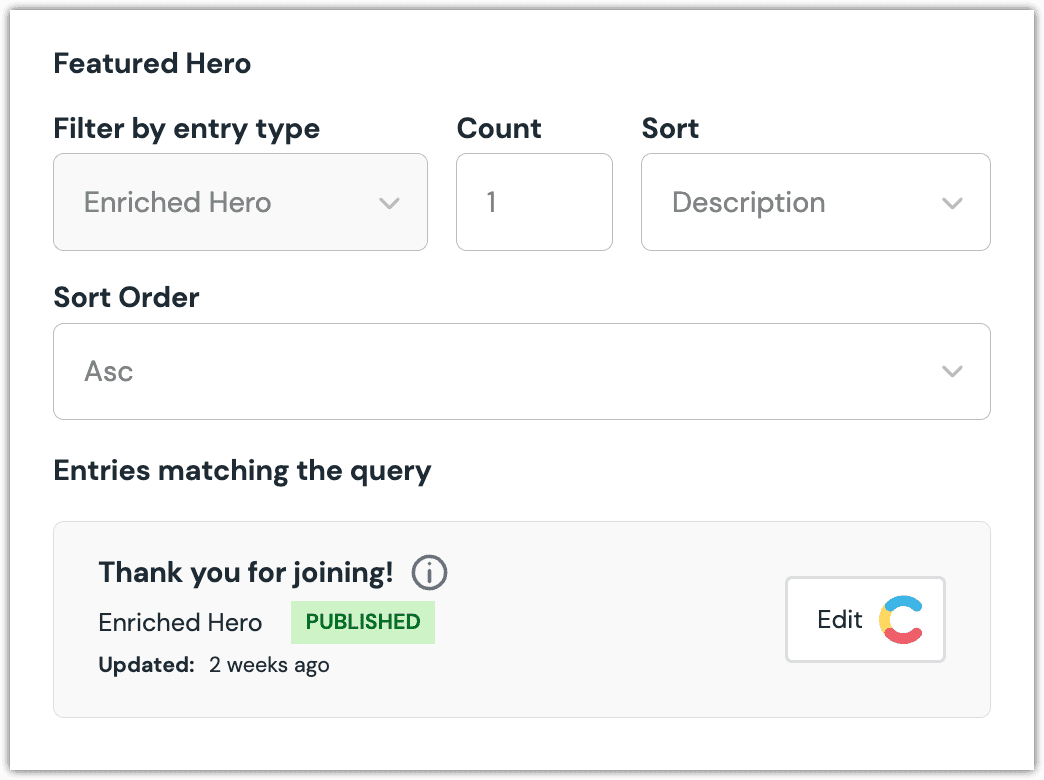
Configure an enhancer#
When an entry query is configured, Uniform only stores the search criteria, not the results of the search. Your front-end application must perform the search using that criteria. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentful.
How Uniform stores the selected settings#
The following is an example of what Uniform stores for the parameter.
There are three identifiers in this value:
- The content type to use in the search.
- The maximum number of entries to search for.
- The source key for the linked content source.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentful to retrieve data for entries.
tip
For more information about the enhancer, see the package documentation.
In Contentful, create a content delivery access token for the enhancer to use to make API calls to Contentful.
Add the following npm packages to your front-end application:
contentful @uniformdev/canvas @uniformdev/canvas-contentfulIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createContentfulEnhancer, ContentfulClientList, CANVAS_CONTENTFUL_QUERY_PARAMETER_TYPES, } from '@uniformdev/canvas-contentful'; import { createClient } from 'contentful';Add the following code:
const client = createClient({ space: '!!! YOUR CF SPACE ID !!!', environment: '!!! YOUR CF ENVIRONMENT NAME !!!', accessToken: '!!! YOUR CF ACCESS TOKEN !!!', });About this step
We recommend you moving the Contentful credentials to environment variables rather than hard-coding them in the front-end app.
Add the following code:
const clientList = new ContentfulClientList({ client }); const contentfulEnhancer = createContentfulEnhancer({ client: clientList })Add the following code:
await enhance({ composition, enhancers: new EnhancerBuilder().parameterType( CANVAS_CONTENTFUL_QUERY_PARAMETER_TYPES, contentfulEnhancer ), context: {}, });
About this step
This registers the enhancer to be used for any occurrence of the Contentful Query parameter.
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentful entries (instead of just being settings).
Override default sort order#
By default, the sort order for the query results is determined by the default Contentful sort order. You can override this using options on the enhancer for the Contentful Query parameter type: