Contentstack parameters
After you add the Contentstack integration to your Uniform project, a number of new parameter types will be available when you configure components using Uniform Canvas.
Parameter type | Description |
---|---|
Contentstack Entry Selector | Select a single entry from Contentstack. |
Contentstack Multi Entry Selector | Select multiple entries from Contentstack. |
Contentstack Query Entry Selector | Specify search criteria that's used to select a single or multiple entries from Contentstack. |
Contentstack Entry Selector#
This parameter type allows a Uniform user to select a single Contentstack entry.
Add parameter to component#
To allow a user to select an entry from Contentstack, you must add a parameter to a component. The parameter is used to store the identifier to the selected entry when the user selects an entry.
In Uniform, navigate to your component.
Add a new parameter using the parameter type Contentstack Entry Selector.
The following values can be specified:
Name Description Allowed Content Types Specifies which content types will be available to select from when the parameter value is edited. You can select multiple content types to allow the user to select from a larger set of entries. Required Indicates whether the value is required when the component is used. Contentstack Entry Selector parameter with a single content type selected.
Edit parameter value#
When you use a component with a Contentstack Entry Selector parameter, by default no entry will be selected. You are prompted to select an entry.
Click Select.
Click the entry you want to select.
About this step
A couple of filters are available. The dropdown allows you to filter by content type. The text box allows you to filter by entry title.
Click Accept to save your selection.
You will see details about the entry you selected, including the title and some metadata.
About this step
After your selection is saved, you are presented with the following options:
- If you want to edit the selected entry in Contentstack, click Edit.
- If you want to deselect the entry, click Unlink.
Configure an enhancer#
When an entry is selected, Uniform only stores the identifier for the entry. Your front-end application must retrieve the details for the entry. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentstack.
How Uniform stores the selected entry#
The following is an example of what Uniform stores for the parameter.
There are two identifiers in this value:
- The entry that was selected.
- The content type for the selected entry.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentstack to retrieve data for entries.
In Contentstack, create an API key and delivery token for the enhancer to use to make API calls to Contentstack.
Add the following npm packages to your front-end application:
npm install contentstacknpm install @uniformdev/canvasnpm install @uniformdev/canvas-contentstackIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements (highlighted lines represent Contentstack specific additions):
import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import contentstack from 'contentstack'; import { createContentstackEnhancer, CANVAS_CONTENTSTACK_PARAMETER_TYPES, } from '@uniformdev/canvas-contentstack';Create an instance of Contentstack enhancer (depending on your region):
const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.US, });const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.EU, });About this step
Please use environment variables here (
process.env.CONTENTSTACK_KEY
for example) instead of hard-coded values.Create an instance of enhancer for Entry parameters:
const entryEnhancer = createContentstackEnhancer({ client: csClient });Register the enhancer for this parameter type with
EnhancerBuilder
. This registers the enhancer to be used for any occurrence of the Contentstack Entry Selector parameter.await enhance({ composition, enhancers: new EnhancerBuilder() .parameterType( CANVAS_CONTENTSTACK_PARAMETER_TYPES, entryEnhancer ), context: {}, });
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentstack entry (instead of just being identifiers).
Contentstack Multi Entry Selector#
This parameter type is added and edited the same way as Contentstack Entry Selector. When editing the parameter value, you can select additional entries by clicking the button Select new.
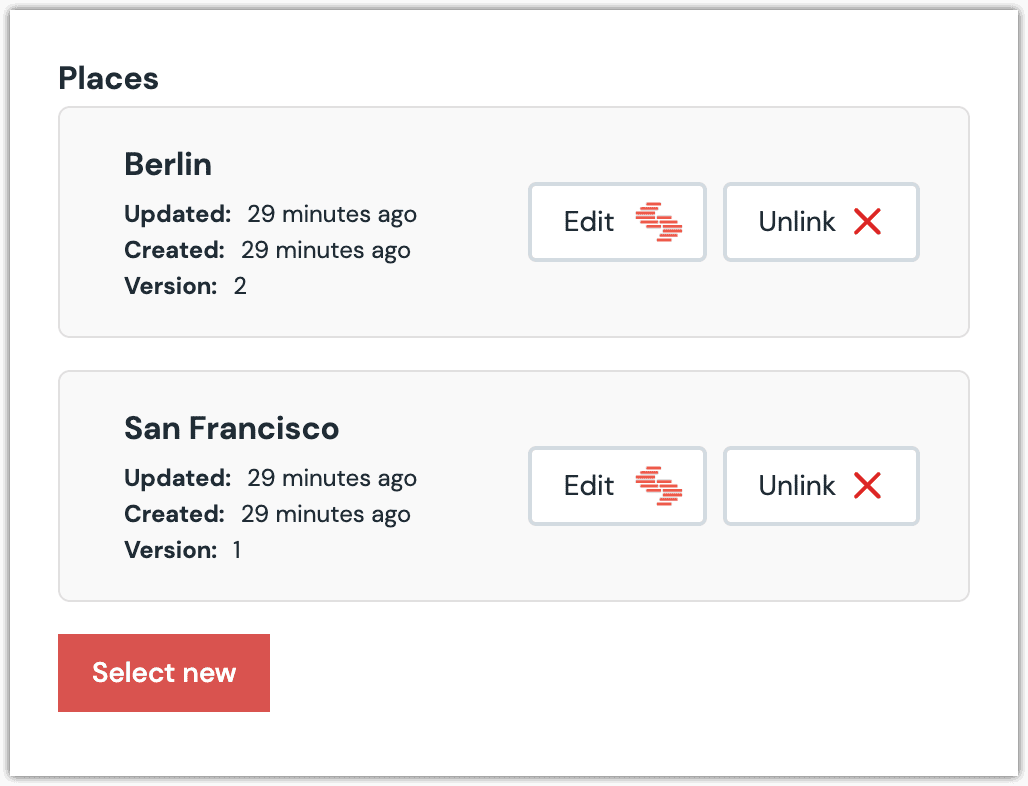
Configure an enhancer#
When entries are selected, Uniform only stores the identifiers for the entries. Your front-end application must retrieve the details for the entries. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentstack.
How Uniform stores the selected entries#
The following is an example of what Uniform stores for the parameter.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentstack to retrieve data for entries.
In Contentstack, create an API key and delivery token for the enhancer to use to make API calls to Contentstack.
If you haven't already, add the following npm packages to your front-end application:
npm install contentstacknpm install @uniformdev/canvasnpm install @uniformdev/canvas-contentstackIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements, notice we import a different enhancer function
createContentstackMultiEnhancer
this time:import contentstack from 'contentstack'; import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createContentstackMultiEnhancer, CANVAS_CONTENTSTACK_MULTI_PARAMETER_TYPES } from '@uniformdev/canvas-contentstack';Create an instance of Contentstack client (depending on your region):
const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.US, });const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.EU, });About this step
Please use environment variables here (
process.env.CONTENTSTACK_KEY
for example) instead of hard-coded values.Create an instance of the multi enhancer by passing the
client
into it:const multiEntryEnhancer = createContentstackMultiEnhancer({ client: csClient });Add
contentstackMultiEnhancer
toEnhancerBuilder
. This step registers the enhancer to be used for any occurrence of the Contentstack Multi Entry Selector parameter:await enhance({ composition, enhancers: new EnhancerBuilder() .parameterType( CANVAS_CONTENTSTACK_MULTI_PARAMETER_TYPES, multiEntryEnhancer ), context: {}, });
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentstack entries (instead of just being identifiers).
Contentstack Query Entry Selector#
This parameter type allows a Uniform user to configure a query that will determine the Contentstack entries that are retrieved.
Add parameter to component#
This parameter type is added the same way as Contentstack Entry Selector.
Edit parameter value#
Configuring this parameter type involves specifying the way a product search should work. You are able to control the following search settings:
Field | Description |
---|---|
Filter by entry type | Only entries that match the selected entry type are included. |
Count | The maximum number of entries that are returned. |
Sort | The entry field used to sort the entries that are returned. |
Sort Order | Whether the entries should be sorted in ascending or descending order. |
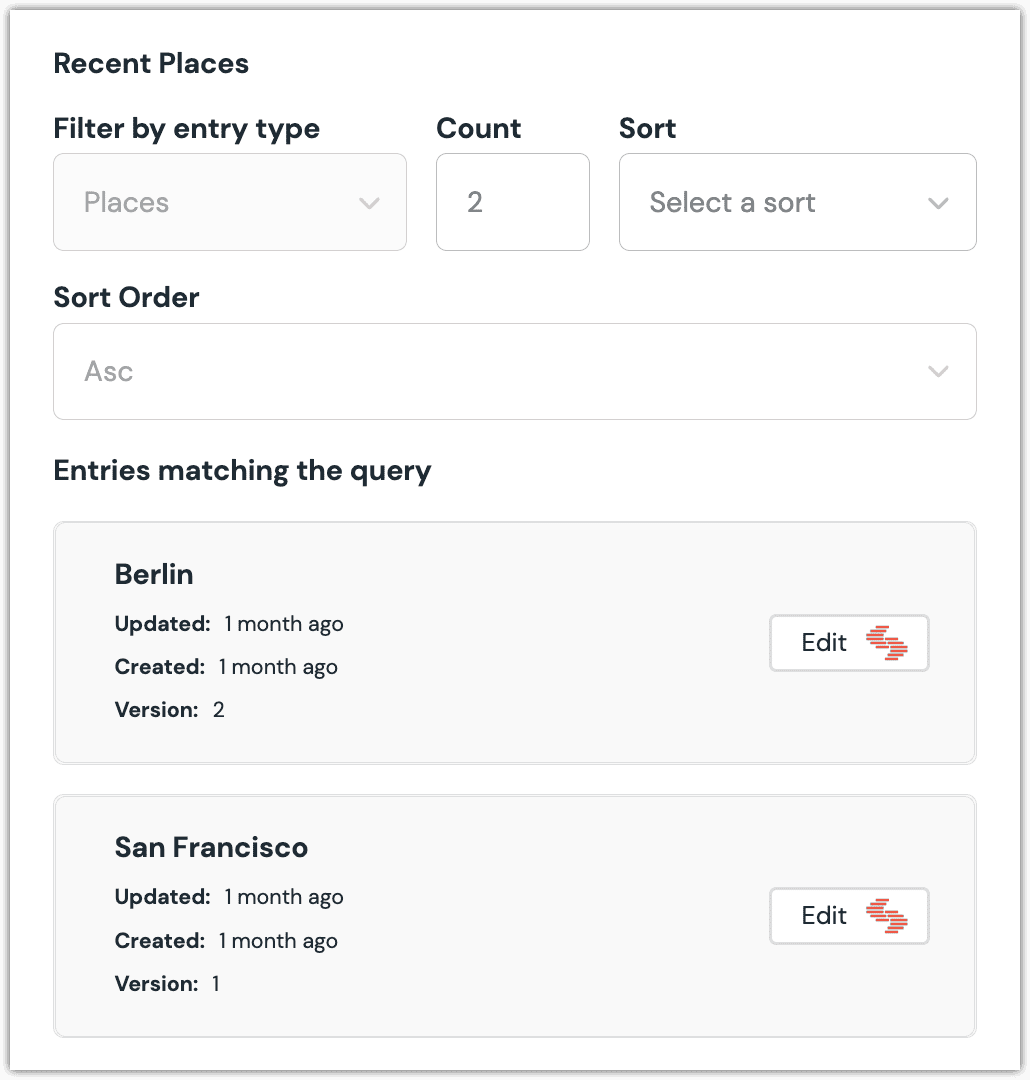
Configure an enhancer#
When an entry query is configured, Uniform only stores the search criteria, not the results of the search. Your front-end application must perform the search using that criteria. Uniform provides an enhancer to simplify this process.
tip
Using the enhancer provided by Uniform saves you from having to write logic to interact directly with Contentstack.
How Uniform stores the selected settings#
The following is an example of what Uniform stores for the parameter.
There are three identifiers in this value:
- The content type to use in the search.
- The maximum number of entries to search for.
- The sort order applies to the search results.
Add the enhancer#
Uniform provides an enhancer so you don't need to write the API calls to Contentstack to retrieve data for entries.
In Contentstack, create an API key and delivery token for the enhancer to use to make API calls to Contentstack.
If you haven't already, add the following npm packages to your front-end application:
npm install contentstacknpm install @uniformdev/canvasnpm install @uniformdev/canvas-contentstackIn a text editor, open the file where you retrieve the composition definition from Uniform.
About this step
You are looking for the code calls the async function
getComposition
. The code below assumes the object returned is set in a variablecomposition
.Add the following import statements:
import contentstack from 'contentstack'; import { EnhancerBuilder, enhance } from '@uniformdev/canvas'; import { createContentstackQueryEnhancer, CANVAS_CONTENTSTACK_QUERY_PARAMETER_TYPES, } from '@uniformdev/canvas-contentstack';Create an instance of Contentstack client (depending on your region):
const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.US, });const csClient = contentstack.Stack({ api_key: '!!! YOUR CONTENTSTACK API KEY !!!', delivery_token: '!!! YOUR CONTENTSTACK DELIVERY TOKEN !!!', environment: '!!! YOUR CONTENTSTACK ENVIRONMENT !!!', region: contentstack.Region.EU, });About this step
Please use environment variables here (
process.env.CONTENTSTACK_KEY
for example) instead of hard-coded values.Create an instance of enhancer for Query parameters:
const queryEnhancer = createContentstackQueryEnhancer({ client: csClient });Register this enhancer with
EnhancerBuilder
:await enhance({ composition, enhancers: new EnhancerBuilder() .parameterType( CANVAS_CONTENTSTACK_QUERY_PARAMETER_TYPES, queryEnhancer ), context: {}, });About this step
This registers the enhancer to be used for any occurrence of the Contentstack Query Entry Selector parameter.
Next steps
Now the parameter value in the composition is mutated to include the field values for the selected Contentstack entries (instead of just being settings).